Node.js 22 · Release Introducing New Set Handling Methods
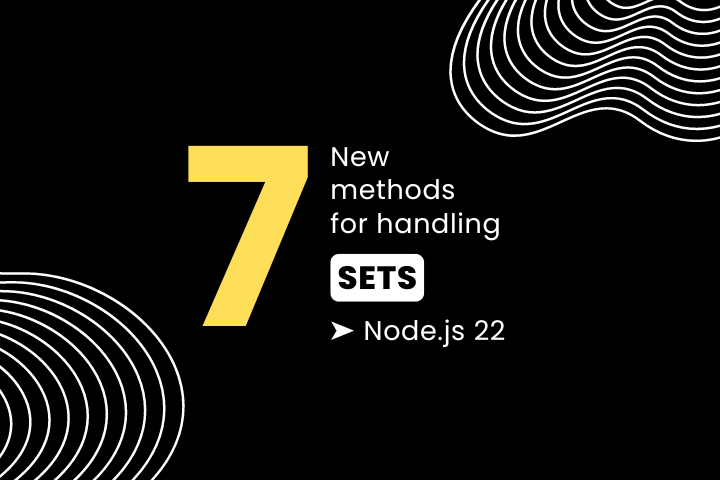
It's official: Node.js 22 has been released and is available since April 24, 2024.
(you can dive directly into the code introducing these methods: here)
Node.js 22 - Notable Changes
The main new features include:
- A built-in WebSocket client
- The
--watch
flag, which enables hot reloading of monitored files, has been moved from an experimental to a stable version, providing a native alternative to nodemon. - Synchronous import of ECMAScript modules (ESM) using the
require()
function. - ... and various additional changes are detailed in the changelog
However, the focus of this post lies in:
- The upgrade of the V8 engine to version 12.4.
This version of the JavaScript runtime engine brings 7 new methods to Set
data structure.
Why Focus on This Upgrade?
Unlike languages like Java or Python, Sets
are not commonly used in JavaScript. Hence, the introduction of new methods for conducting fundamental mathematical operations on this data type might incentivize developers to employ Sets
more extensively.
This development was supported by TC39, the technical committee of ECMA International, responsible for standardizing the language.
The comprehensive work can be accessed in their Github repository: proposal-set-methods. The initial discussions on this topic trace back to May 2018.
Hands-on Examples of New Set Methods
Let's go into the details and provide practical examples to illustrate the new methods available in the Set
object.
1. union
The union
method merges two separate sets
into a single Set
that includes all the unique elements from both original sets
.
const setA = new Set(["🐉", "🦄", "🔥"]);
const setB = new Set(["🐎", "🦅", "🐺"]);
console.log(setA.union(setB));
// Set(6) { "🐉", "🦄", "🔥", "🐎", "🦅", "🐺" }
More details on the MDN Web Docs : Set.prototype.union().
2. intersection
The intersection
method finds the elements that exist in both distinct sets
and gathers them into a new Set
.
const setA = new Set(["🐉", "🦄", "🔥"]);
const setB = new Set(["🐎", "🦅", "🐉"]);
console.log(setA.intersection(setB));
// Set(1) { "🐉" }
More details on the MDN Web Docs : Set.prototype.intersection().
3. difference
The difference
method identifies and retrieves elements present in one Set
but absent from another supplied Set
.
const setA = new Set(["🐉", "🦄", "🔥"]);
const setB = new Set(["🐉", "🦅", "🐎"]);
console.log(setA.difference(setB));
// Set(2) { "🦄", "🔥" }
More details on the MDN Web Docs : Set.prototype.difference().
4. symmetricDifference
The symmetricDifference
method finds elements present in one Set
or the other, but not in both sets
simultaneously.
const setA = new Set(["🐉", "🦄", "🔥"]);
const setB = new Set(["🐉", "🦅", "🐎"]);
console.log(setA.symmetricDifference(setB));
// Set(4) { "🦄", "🔥", "🦅", "🐎" }
More details on the MDN Web Docs : Set.prototype.symmetricDifference().
5. isSubsetOf
The isSubsetOf
method verifies whether all elements of a Set
are also present in another Set
.
const setA = new Set(["🐉", "🦄"]);
const setB = new Set(["🐉", "🦄", "🔥", "🦅"]);
console.log(setA.isSubsetOf(setB)); // true
More details on the MDN Web Docs : Set.prototype.isSubsetOf().
6. isSupersetOf
The isSupersetOf
method verifies whether all elements of a given Set
are included in the current Set
.
const setA = new Set(["🐉", "🦄", "🔥", "🦅"]);
const setB = new Set(["🐉", "🦄"]);
console.log(setA.isSupersetOf(setB)); // true
More details on the MDN Web Docs : Set.prototype.isSupersetOf().
7. isDisjointFrom
The isDisjointFrom
method checks whether two sets
have no elements in common.
const setA = new Set(["🐉", "🦄"]);
const setB = new Set(["🔥", "🦅"]);
console.log(setA.isDisjointFrom(setB)); // true
More details on the MDN Web Docs : Set.prototype.isDisjointFrom().
Conclusion
Here's a summary of the new methods for Sets
, designed to enhance their functionality. Will they lead to wider adoption by developers?
Only time will tell... 👀
Additional Notes
This article focuses on the availability of methods in Node.js. However, it's important to mention that these methods were available in browsers like Chrome, Opera, and Safari a bit earlier:
- Chrome and Edge: v122 - February 2024
- Opera: v108 - March 2024
- Safari: v17 - September 2023
Focus on Your Clients: Let Us Handle Your Code
Shift your focus to serving your clients while we handle your source code.
Explore cycaas.com to discover our suite of services, from code review to refactoring, allowing you to deliver top-notch solutions without distraction.